Spring Boot is a popular, open-source, enterprise-level framework for creating standalone, production-grade applications that run on the Java Virtual Machine (JVM).
It requires minimal or zero configuration and is easy to get started. It is a widely popular choice among Java developers for developing MicroServices and web applications.
Spring boot is built on top of the popular Spring Framework and inherits features like dependency injection(DI) or Inversion of Control (IoC) from the Spring.
It offers built-in support for typical tasks that an application needs to perform, such as data binding, type conversion, validation, exception handling, resource and event management, internationalization, and more.
Why Spring Boot when we have Spring?
While the Spring framework is a powerhouse with many built-in production-ready features, it requires complex configurations. We need to create XML or Java-based configurations to enable different features of the Spring Framework.
While we can use Spring Framework for building Microservices, it doesn't provide any specific tools and features to support Microservices architecture. Traditionally, the Spring applications are deployed to an external application server such as Tomcat or JBoss. However, the Spring boot framework makes it easy to deploy Microservices using the built-in Embedded Servers.
In the Spring framework, developers need to manage dependencies manually, which can sometimes lead to version conflicts and other issues.
What can we do with Spring Boot?
Spring Boot framework provides several features that make it ideal for building a variety of applications, including:
- You can create complex multi-tier web applications that can serve millions of users.
- Spring Boot makes it easy to create RESTful APIs to expose your data and functionality to other applications.
- Widely used choice for building small, independent services that can be easily scaled and deployed.
- You can build a Spring batch application that can process large volumes of data based on a specific schedule or process based on a specific event.
- Spring Boot also can be used to build applications that run on the command line.
Key Features of Spring Boot
Some of the key features of the Spring Boot framework are:
- Auto-configuration
- Dependency Injection (DI)
- Spring Boot Starter Dependencies
- Embedded Server
- Production-ready features
Auto Configuration
Auto-configuration is a feature in the Spring Boot Framework that aims to simplify the setup and configuration of Spring applications.
It makes it easier to create production-ready applications with minimal effort. It automatically configures the application based on the dependencies present in the classpath.
When you include certain libraries or dependencies in your Spring Boot project, the framework automatically configures beans, settings, and components to provide a set of defaults. This means you don't need to do any manual configuration, as Spring Boot will set up the application based on conventions and best practices.
For example:
If your project includes the Spring Data JPA dependency in your pom.xml or build.gradle
file then the Spring Boot framework will automatically configure a DataSource
bean, an EntityManager
bean for you to work with JPA.
If we want to customize the data source configuration, then we can override the following properties in the application.properties
file to connect to a different data source.
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=myuser
spring.datasource.password=mypassword
Auto-configuration helps developers reduce the number of errors caused due to misconfiguration and makes developers more productive.
Dependency Injection
The Dependency Injection(DI) is one of the core features of the Spring framework. This allows developers to write loosely coupled code that is easy to maintain and test.
It is a software design pattern used in object-oriented programming, where the dependencies of a class are provided by an external entity rather than being created within the class itself. In other words, instead of a class creating its dependencies, those dependencies are "injected" into the class from outside.
The DI concepts are discussed more in detail in Chapter 4.
Spring Boot Starter Dependencies
For developing web applications or Micro-services, you will be required to add multiple external libraries for your application. For example, if you want to have logging support, you would need Logback or Log4J dependency.
Traditionally, we used to download the jar files and include into your project, but with build tools like Gradle and Maven, managing the dependencies becomes easy. However, the build tools do not solve the version compatibility issue.
For example, If Lib1 depends on Lib2, you need to include both libraries manually in your pom.xml
or build.gradle
file and also you need to be careful about the version compatibility of each of those libraries. If Lib1 is compatible with a specific version of Lib2, then you need to be aware of that and include the compatible versions of Lib2 in your dependency descriptor file.
As your application grows, the number of dependencies will grow and managing those dependencies manually is very cumbersome and error-prone.
That’s where the Spring boot starter packages come to the rescue. Spring Boot provides us with several Spring Boot starter packages to address this problem.
There are two types of dependencies:
- Direct: These are dependencies defined in your
pom.xml
orbuild.gradle
file under thedependencies
section. - Transitive: These are dependencies that are dependencies of your direct dependencies.
For example, if we add the spring-boot-starter-web
as a dependency to your pom.xml
file then will download spring-boot-starter-tomcat
as the direct dependency and with that it will also download the other transitive dependencies like tomcat-embedded-core
and tomcat-embedded-el
and tomcat-embedded-websocket
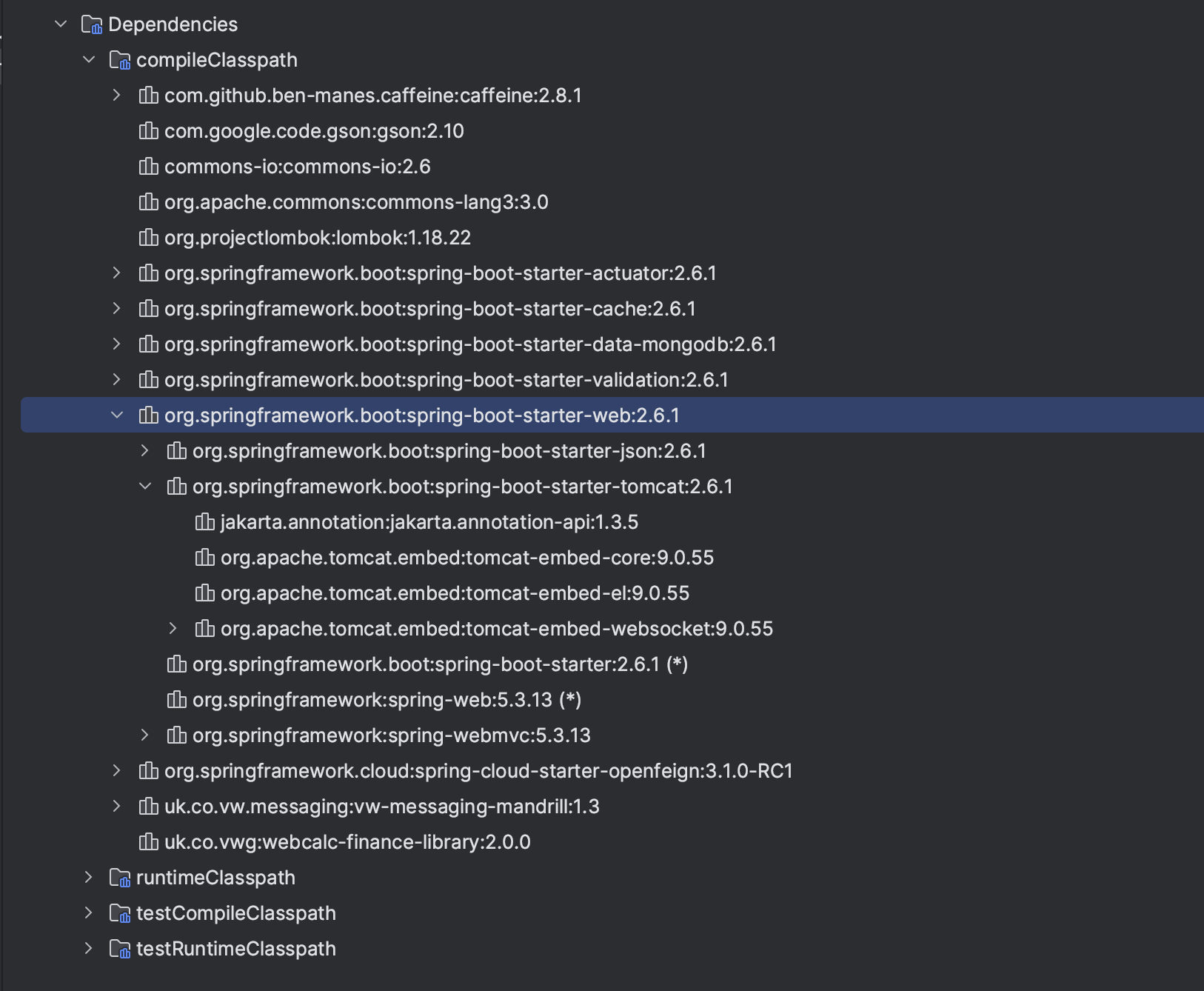
If you’re managing the dependencies manually, then you must be very careful about the specific version of the dependency you are using and also need to worry about their compatibility with each other.
Starter dependencies are a set of convenient dependency descriptors that we can use to bootstrap our Spring boot applications. They contain a lot of pre-defined dependencies with a supported set of transitive dependencies.
The starter dependencies ensure all the direct and transitive dependencies are always compatible with each other and you don't have to worry about managing those versions manually and focus only on application development.
Spring Boot starters follow a similar naming pattern, e.g. spring-boot-starter-XYZ
, where XYZ denotes a particular type of library.
Here are some of the popular Spring Boot starter packages:
spring-boot-starter-web | It is used for building web applications, including RESTful applications using Spring MVC. It uses Tomcat as the default embedded container |
spring-boot-starter-activemq | It is used in JMS messaging using Apache ActiveMQ. |
spring-boot-starter-actuator | Provides production-ready features such as health checks, monitoring, and other application management features. |
spring-boot-starter-batch | It is used for the Spring Batch. |
spring-boot-starter-cache | It is used for Spring Framework's caching support. |
spring-boot-starter-data-jpa | It is used for Spring Data JPA with Hibernate. |
spring-boot-starter-data-mongodb | It is used for MongoDB document-oriented database and Spring Data MongoDB. |
spring-boot-starter-mail | It is used to support Java Mail and Spring Framework's email sending. |
Embedded Servers
Spring Boot includes support for embedded Tomcat, Jetty, and Undertow servers. This means you don’t need any external web servers and no need to deploy WAR files anymore.
The default embedded server is Tomcat, and it is available through the spring-boot-starter-web
dependency. If you want to use the Jetty or Undertow server instead of Tomcat, then you can include spring-boot-starter-jetty
or spring-boot-starter-undertow
dependencies.
Checkout the article that explains how to replace default embedded Tomcat Server with Jetty or Undertow.
Production Ready Features
Spring Boot Framework includes production-ready features such as metrics, health checks, and external configuration management. Spring Boot also provides integration with various enterprise technologies such as RMI, JPA and JMS, AMQP, etc.
- RMI
- Hibernate
- WebSocket API (JSR 356)
- AMQP - Advanced Message Queuing Protocol
- Java Web Services
- JPA (JSR 338) - Java Persistence API
- JMS
Spring also provides a Model-View-Controller (MVC) framework that simplifies the development of web applications by separating the presentation layer from the business logic.
It also provides several tools and frameworks for testing applications, including the Spring Test Framework and the Spring MVC Test Framework.
Spring Boot vs. Spring: What Works for You?
Ultimately, the best framework for you will depend on your specific requirements. If you are not sure which framework to choose, I recommend starting with Spring Boot.
Features | Spring | Spring Boot |
---|---|---|
Source type | It is an open-source framework. | It is built on top of a spring. |
Core features | Dependency injection | Auto-configuration is an essential feature. |
Deployment | The server is set explicitly for spring. The deployment descriptor is required. | Embedded servers are provided. The deployment descriptor is not required. |
Use cases | Good for complex applications. | Good for rapid application development |
Setup time | It takes more time to set up. | Faster to set up. |
Spring Boot is a great choice to get started with Spring development without having to worry about the complexities of the Spring Framework. Once you understand Spring better, you can decide if you need the additional features and flexibility of the Spring Framework.