Before we begin, we need the following prerequisites for developing Spring Boot applications.
- Java 17 or 21
- IDE (Integrated Development Environment):
Java 17 or 21
The current stable version of the Spring boot framework is 3.2.0, and it requires Java17 and is compatible up to and including Java 21.
Check if you have Java installed using the following command.
java --version
If Java is not installed, you can follow the installation process from my blog post How to Install Java for MacOS and Windows?
IDE (Integrated Development Environment):
An Integrated Development Environment (IDE) is a tool that helps to streamline the coding, debugging, and project management for developers. It offers features like code editing, refactoring, debugging, integrated terminal, and many other features to support application development.
- VSCode - Free
- Eclipse - Free
- IntelliJ IDEA Community (Free) or IntelliJ Ultimate (Licensed)
I prefer IntelliJ IDEA, as it is one of the most advanced and preferred IDEs for Java developers. If you haven’t installed it already, you can install the community edition of IntelliJ here https://www.jetbrains.com/idea/download/
Choosing build tools Maven vs. Gradle
Build tools play a crucial role in software development. Build tools are used to automate the process of compiling, testing, packaging, and deploying your application. This can save you a lot of time, especially when working with a large, distributed team.
Using build tools you can also simplify the process of managing dependencies for your project. It helps to automate the process of resolving and including external libraries in your project.
Primarily there are two popular build tool choices for Spring Boot:
- Maven
- Gradle
Maven:
Maven is a popular build automation tool used
primarily for Java projects. It uses an XML file called a pom.xml
(Project Object Model)
to define the project's configuration.
The pom.xml
file serves as the central
configuration file for a Maven project. It contains information about the project, including its
dependencies, plugins, build lifecycle, and other relevant details.
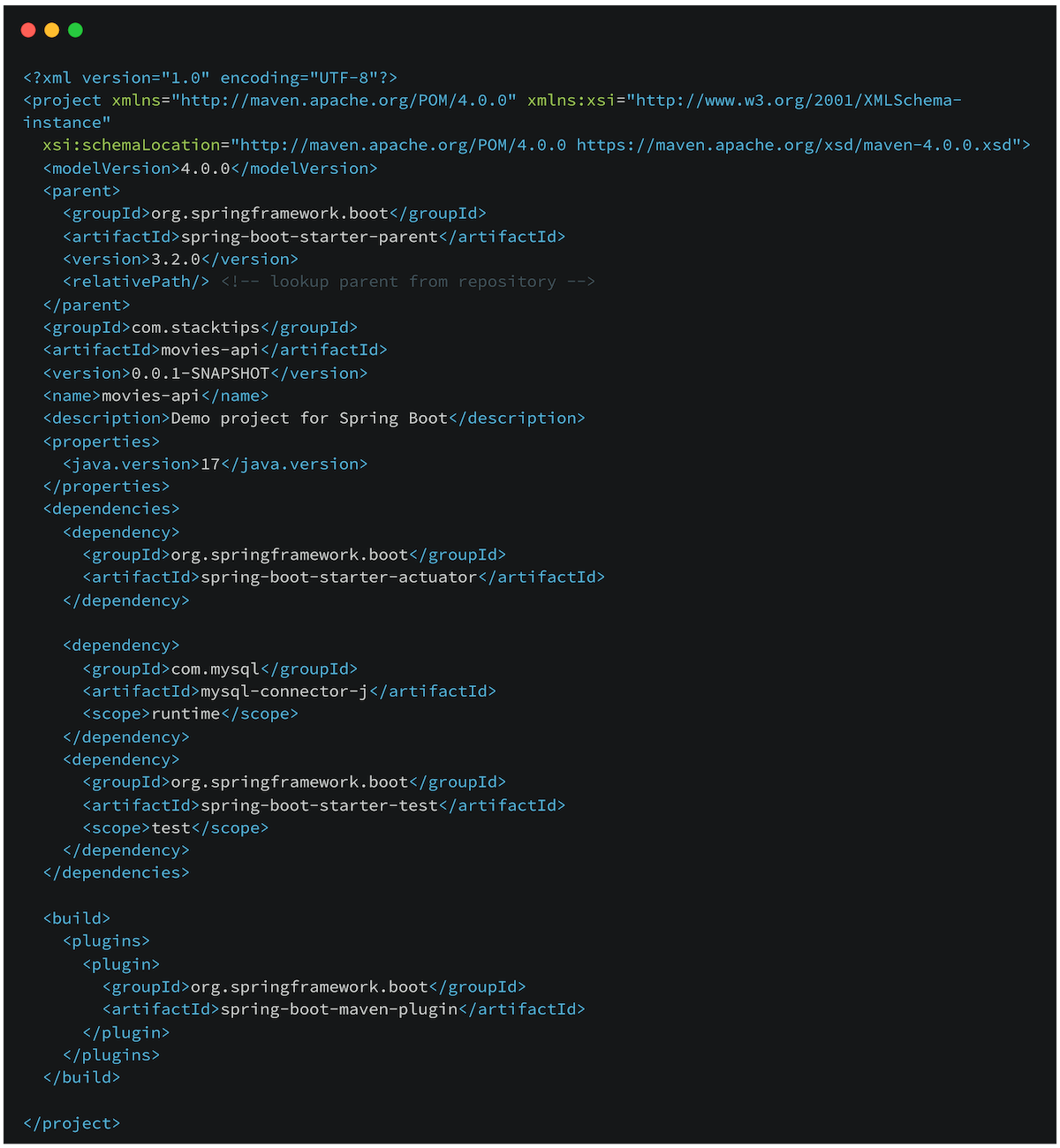
Gradle
Gradle is another build automation tool that uses
either Groovy or Kotlin DSL (Domain-Specific Language) for defining build scripts. Gradle maintains the
dependency configurations in build.gradle
file and it is typically stored in the
project's root directory.
An example of a Gradle build file for a Spring Boot application follows.
Choosing Between Maven and Gradle
The choice between Maven and Gradle for Spring Boot development depends on your personal preferences and the specific needs of your project.
While Maven is more mature and almost like an established standard in the Java community, Gradle is a more modern build system and has gained popularity recently. Both Gradle and Maven are very powerful and good fit for larger and more complex projects.
Create a New Spring Boot Project
There are a few convenient options available for bootstrapping your Spring Boot project template.
- Using Spring Initializr
- Using the Spring Boot CLI
- Using IntelliJ
Using Spring Initializer
Spring initializer is a web-based tool that helps you to configure and generate the Spring boot project template. Let us use the Spring initializer to create a new Spring Boot project.
First, visit https://start.spring.io in your browser to open Spring initializer.
Let us now configure our project:
- Project Type: Select the Maven or Gradle build type and select the language. Let’s select Gradle with Java.
- Language: You can write Spring Boot code using Java, Kotlin, or Groovy. We will use Java throughout this course.
- Spring Boot Version: Select the Spring Boot version out of all available versions. At the time of creating this video Spring Boot 3.2.0 is the latest release version. Let’s select that
Now, provide the basic project details like the group, artifact, and name of your project.
- Group: Usually the reverse of your domain. If your
domain is stacktips.com, your group name will be
com.stacktips
- Artifact Id: The artifact name is the name of the jar
without a version. For example, if your artifact name is
movies-api
, then the jar will bemovies-api-[version].jar
. - App Name: Provide a name for your application. Let’s
call it a
movies-api-app
. - Description: You may additionally describe your project.
- Package name:This is the
base package name for your project. Let’s use
com.stacktips.app
as our package name. - Package type: We have two choices for the package type, Jar and WAR. Let us select Jar
💡War vs Jar:
Both JAR (Java Archive) and WAR (Web Archive) are formats used in the Java ecosystem for packaging and distributing applications. But they have a completely different purpose.
JAR (Java Archive):
- Jar files are used to package and distribute standalone Java applications. They contain a set of classes, resources, and other files that are required to run the application.
- JAR files are typically self-contained, meaning that they do not require any additional dependencies to be installed on the system where they are run.
WAR (Web Archive):
- WAR files are a specific type of JAR file that is used to package and distribute web applications.
- They contain all of the files that are required to run the web application, including the Java classes, web pages, static resources, and deployment descriptor files.
- WAR files are typically deployed to a web server, where they are run in a servlet container.
- Java Version: Spring boot 3.2.0 require minimum of Java 17 and it is compatible up to Java 21. I have Java 21 installed in my system, so I have selected Java 21, but you can very well choose Java 17.
- Select Starter Dependencies: On the right-hand side, you will notice the Add dependency button. Clicking on the Add dependencybutton will allow you to choose the Spring boot starter dependencies for your project. Let us for now choose Spring Boot Starter Web and Spring Boot Actuator for this example.
- Explore and Generate: Once all details are filled in, you can click on the Explore button to see the project structure beforehand. Once you’ve verified your configurations, you can click on Generate button.
This will download a new Spring Boot project with the configuration you’ve selected. Now, you can extract the zip file and open the project in your IntelliJ IDEA.
Creating Spring Boot Project using Spring CLI
The Spring Boot CLI (Command Line Interface) is a command line tool that can be used to generate the spring boot project. Everything you can do with the Spring initializer can be configurable though Spring Boot CLI.
Before we work the Spring Boot CLI we need to ensure the CLI is installed and available for us to use. To check if the Spring Boot CLI is installed, type the following command:
spring --version
If it is installed, you will see the Spring Boot version printed as follows
Spring CLI v3.2.0
If is is not installed, there are several ways we can install the Spring CLI. We can install using SDKMAN, OSX Homebrew, MacPorts or using manual installation methods. The scope of this chapter covers the installation using SDKMAN, but if you prefer other installation methods, then you may refer the official installation guide.
Installing the Spring Boot CLI
Install Spring Boot CLI using SDKMAN
Before we install, let us first get the list of available Spring Boot releases using the following command.
sdk ls springboot
It will print all the list of available Spring Boot releases.
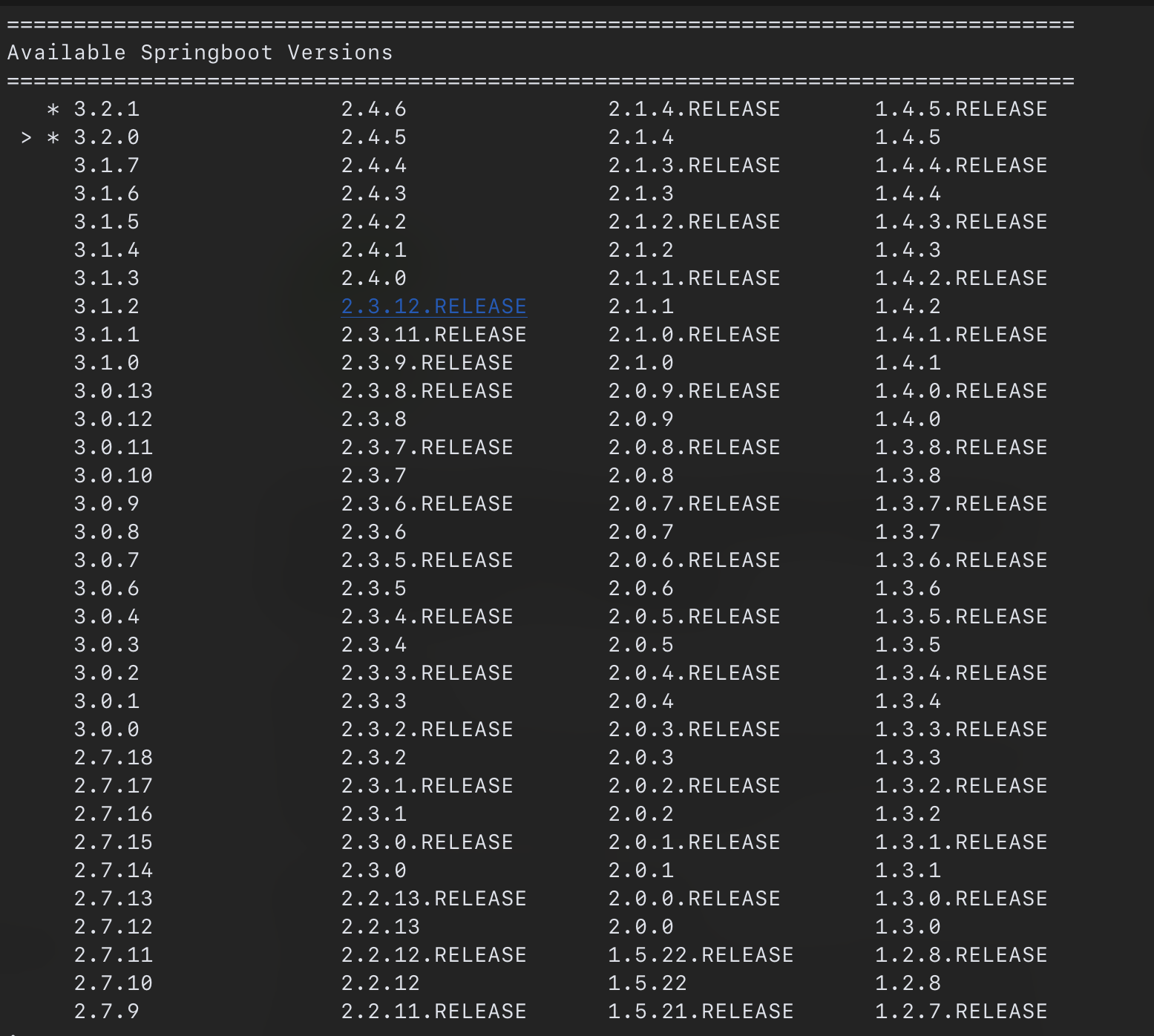
To install Spring Boot CLI,
sdk install springboot
Once Spring Boot CLI is installed, You can list the
capabilities of the service by using the --list
flag:
spring init --list
Now to Create the Spring Boot project, we can use the followinng command;
spring init --type=gradle-project
--java-version=17
--language=java
--name=movies-api
--artifact-id=movies-api
--group-id=com.stacktips
--packaging=jar
--dependencies=web,actuator
movies-api-app
This command will create a Spring Boot project inside
the movies-api-app
directory. All the folders and files created is similar to the one we
have created using the Spring Initilizer.
You do not need to use the Spring CLI to work with Spring Boot, but it is a quick way to get a Spring application off the ground without using Spring Initilizer.
Using IntelliJ
You can also create a Spring Boot project from IntelliJ, but for that, you will need the IntelliJ Ultimate edition which requires a commercial license.
Build and Run Spring Boot Project
Before we build and run our code, let us first open the IntelliJ IDE and import the project we have created. To import the project:
- Go to File | Open.
- And browse the downloaded folder and click on Open button. your IDE, then it may take a while as it has to download all the direct and transitive dependencies as configured for your project. The load time will be based on your internet speed. There are several different ways we can build and run a Spring Boot project. Some of the most common methods are:
- Running from IDE
- Using Gradle or Maven build tools
- Run as a Far JAR
Running from an IDE
This is the simplest way to run a Spring Boot project. Every IDE is equipped with options that allow developers to build and run the spring boot application in a single click.
Open the MoviesApiApplication
class with
the main()
method (it is usually also designated with
the @SpringBootApplication
annotation), click in the gutter, and select to run the
class.
Build and Run using Gradle Command
For Gradle projects, you can build the Spring boot project to into an executable Jar using the following command:
./gradlew build
Start/Run spring boot application
./gradlew bootRun
Build and Run using Maven Command
For Maven projects, you can build the Spring boot project to into an executable Jar using the following command:
./mvnw clean package
Start/Run spring boot application
./mvnw spring-boot:run
Running Application as a FatJar
To run the application as a FatJar we first need to package the Spring Boot application into an executable Jar file. For that, we can use a build tool like Maven or Gradle.
Maven:
./mvnw clean package
Gradle:
./gradlew clean build
Once the jar files are built, we can run the
application as a fat jar using the java -jar
command.
java -jar build/libs/movies-api-0.0.1-SNAPSHOT.jar
When you run your Spring Boot application, you will see a log message like this:
Notice that we are using mvnw
and gradlew
wrapper
utilities to build and package our Spring boot application. We will learn more about them in the
next chapter.